Woking with Microsoft Graph API.net MVC application
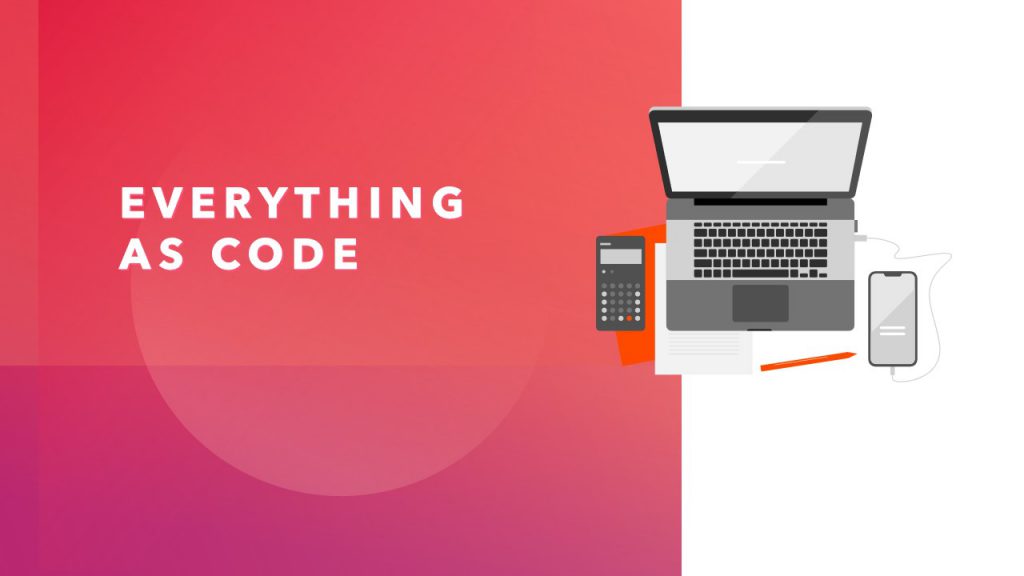
How you can use the Microsoft Graph API to connect to Azure AD and fetch user details in a .NET MVC web application:
First, install Microsoft.Graph NuGet package in your MVC project.
Install-Package Microsoft.Graph
Next, add the following using statements at the top of your controller file:
using Microsoft.Graph;
using Microsoft.Identity.Client;
using System.Threading.Tasks;
Then, create a method that will use the Microsoft Graph API to fetch user details from Azure AD. In this example, we will fetch the user’s display name and email address.
public async Task<IActionResult> GetUserDetails()
{
// Get the access token for the Microsoft Graph API
var accessToken = await GetAccessToken();
// Use the access token to authenticate the Microsoft Graph API request
var graphClient = new GraphServiceClient(new DelegateAuthenticationProvider((requestMessage) =>
{
requestMessage.Headers.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Bearer", accessToken);
return Task.CompletedTask;
}));
// Fetch the user's details from Azure AD
var user = await graphClient.Me.Request().Select("displayName,mail").GetAsync();
// Return the user's display name and email address to the view
return View(user);
}
Finally, you will need to implement the GetAccessToken
method that is used to get an access token for the Microsoft Graph API. This method will use the Microsoft Authentication Library (MSAL) to acquire an access token from Azure AD.
private async Task<string> GetAccessToken()
{
// Your Azure AD app's client ID
string clientId = "your-client-id";
// Your Azure AD app's redirect URI
string redirectUri = "your-redirect-uri";
// Your Azure AD tenant ID
string tenantId = "your-tenant-id";
// The scopes you want to request access to
string[] scopes = new string[] { "User.Read" };
// Create an instance of the MSAL ConfidentialClientApplication class
var app = ConfidentialClientApplicationBuilder.Create(clientId)
.WithRedirectUri(redirectUri)
.WithTenantId(tenantId)
.Build();
// Use MSAL to acquire an access token for the Microsoft Graph API
var result = await app.AcquireTokenForClient(scopes)
.ExecuteAsync();
return result.AccessToken;
}
In the code above, you will need to replace your-client-id
, your-redirect-uri
, and your-tenant-id
with the actual values for your Azure AD app. You can find these values in the app registration page in the Azure portal.
You can then use the GetUserDetails
action method in your MVC controller to fetch the user’s details from Azure AD and display them in your view.