Azure Security Review
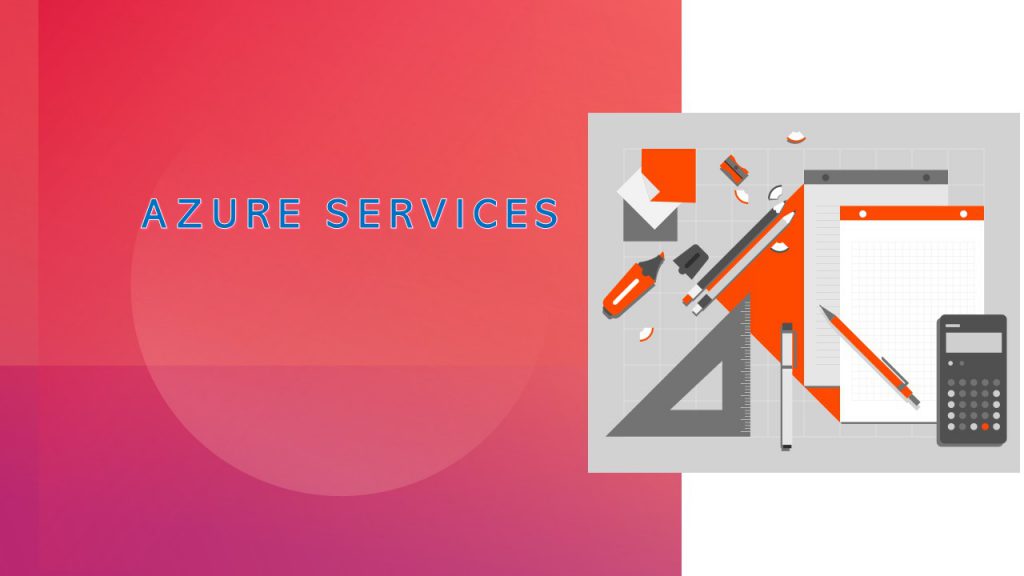
The Most Important Element of Azure security is that you don’t leave any loose ends in your environment.
This script will check for unassigned Network Security Groups (NSGs), open ports on NSGs, unsecured storage accounts, and unsecured access keys.
# Import Azure PowerShell module Import-Module AzureRM # Connect to Azure subscription Connect-AzureRmAccount # Define the Azure subscription to use $subscriptionId = "your_subscription_id" Select-AzureRmSubscription -SubscriptionId $subscriptionId # Check for unassigned network security groups (NSGs) Write-Output "Checking for unassigned NSGs..." $unassignedNSGs = Get-AzureRmNetworkSecurityGroup | Where-Object {-not (Get-AzureRmNetworkSecurityGroupAssociation -NetworkSecurityGroup $PSItem)} if ($unassignedNSGs) { Write-Output "Unassigned NSGs found:" $unassignedNSGs | Select-Object Name, ResourceGroupName } else { Write-Output "No unassigned NSGs found." } # Check for open ports on NSGs Write-Output "Checking for open ports on NSGs..." $openPorts = Get-AzureRmNetworkSecurityGroup | Get-AzureRmNetworkSecurityRuleConfig | Where-Object {$PSItem.Access -eq "Allow" -and $PSItem.Direction -eq "Inbound" -and $PSItem.Priority -lt 65000 -and ($PSItem.SourceAddressPrefix -eq "*" -or $PSItem.SourcePortRange -eq "*")} if ($openPorts) { Write-Output "Open ports found:" $openPorts | Select-Object Name, Priority, Access, Direction, SourceAddressPrefix, DestinationAddressPrefix, SourcePortRange, DestinationPortRange } else { Write-Output "No open ports found." } #Check for unsecured storage accounts Write-Output "Checking for unsecured storage accounts..." $storageAccounts = Get-AzureRmStorageAccount foreach ($storageAccount in $storageAccounts) { $keys = Get-AzureRmStorageAccountKey -StorageAccountName $storageAccount.StorageAccountName if ($keys.Value[0].Permission -ne "Full" -or $keys.Value[1].Permission -ne "Full") { Write-Output "Unsecured storage account found:" $storageAccount | Select-Object StorageAccountName, ResourceGroupName } } #Check for unsecured access keys Write-Output "Checking for unsecured access keys..." $accessKeys = Get-AzureRmStorageAccountKey -StorageAccountName $storageAccount.StorageAccountName foreach ($key in $accessKeys.Value) { if ($key.Permission -ne "Full") { Write-Output "Unsecured access key found:" $key | Select-Object KeyName, Permission } }
You can customize the script to check for other security issues as well.