JavaScript Application to do VM Start-Stop on Azure
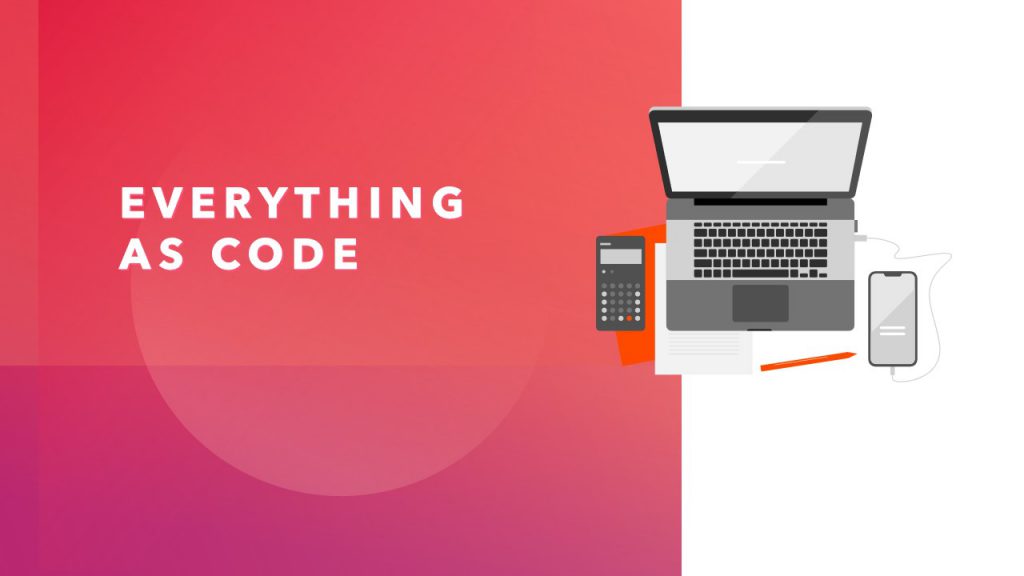
JavaScript application that can be used to fetch the current state of an Azure virtual machine (VM) and display a start or stop button on an HTML page based on its state:
// Import the required libraries
const msRestAzure = require('ms-rest-azure');
const ComputeManagementClient = require('azure-arm-compute');
// Set the subscription ID and resource group name
const subscriptionId = 'your-subscription-id';
const resourceGroup = 'your-resource-group-name';
// Set the name of the Azure VM
const vmName = 'your-vm-name';
// Authenticate using an Azure service principal
msRestAzure.loginWithServicePrincipalSecret('client-id', 'client-secret', 'tenant-id', (err, credentials) => {
if (err) return console.error(err);
// Create a new ComputeManagementClient
const computeClient = new ComputeManagementClient(credentials, subscriptionId);
// Fetch the VM and its current state
computeClient.virtualMachines.get(resourceGroup, vmName, (err, result) => {
if (err) return console.error(err);
// Check if the VM is currently running
if (result.properties.provisioningState === 'Succeeded') {
// Set the button text to "Stop VM"
document.getElementById('vmButton').innerHTML = 'Stop VM';
} else {
// Set the button text to "Start VM"
document.getElementById('vmButton').innerHTML = 'Start VM';
}
});
});
In the code above, you will need to replace your-subscription-id, your-resource-group-name, and your-vm-name with the actual values for your Azure subscription, resource group, and VM.
You will also need to provide the client ID, client secret, and tenant ID of an Azure service principal that has permissions to manage the VM.
When the code is run, it will authenticate using the service principal and then fetch the current state of the specified VM. It will then update the text of the vmButton element on the HTML page with either “Start VM” or “Stop VM” based on the VM’s current state.
Here is an example HTML page that you can use to run the JavaScript code above:
<html>
<head>
<title>Azure VM State</title>
</head>
<body>
<button id="vmButton"></button>
<script src="vm-state.js"></script>
</body>
</html>
In this example, the vm-state.js file contains the JavaScript code shown above. When the HTML page is loaded, it will run the JavaScript code and display a start or stop button on the page based on the current state of the VM.