Working with Azure AD logs with Graph API and PowerShell
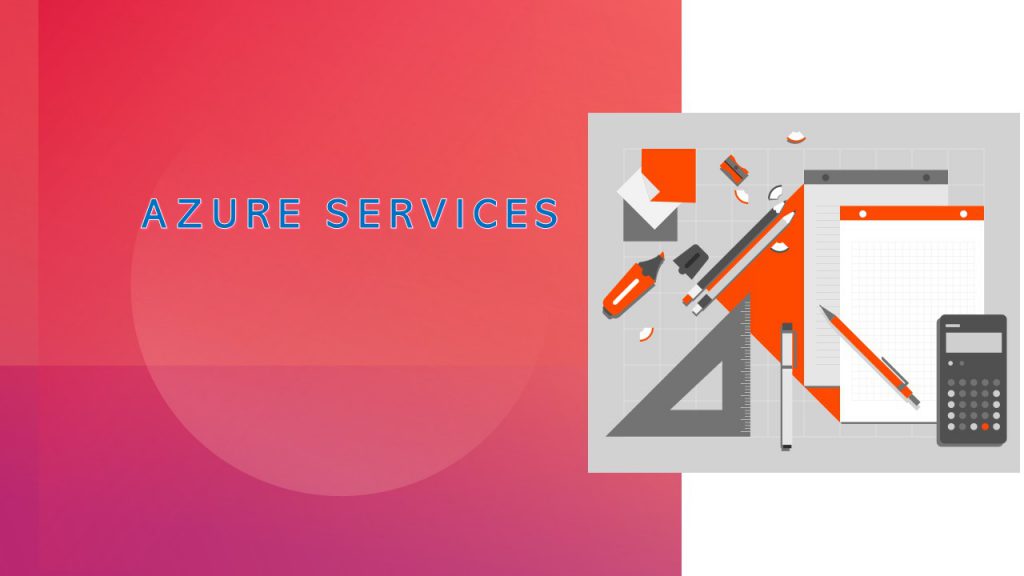
Azure AD logs are a valuable source of information for monitoring and troubleshooting user activities and authentication events in your organization. However, accessing and analyzing these logs can be challenging, especially if you have a large number of users and applications. In this blog post, I will show you how to use the Microsoft Graph API and PowerShell to retrieve and work with Azure AD logs in a simple and efficient way.
The Microsoft Graph API is a unified endpoint for accessing data and insights from various Microsoft cloud services, including Azure AD. The Microsoft Graph PowerShell module is a wrapper around the Graph API that provides cmdlets for interacting with different resources and entities. Using this module, you can easily query and manipulate Azure AD logs without having to deal with complex REST requests and responses.
To get started, you need to install the Microsoft Graph PowerShell module from the PowerShell Gallery:
Install-Module -Name Microsoft.Graph
You also need to register an app in Azure AD and grant it the required permissions to access the audit logs and sign-in logs. You can follow this tutorial to learn how to do that: https://learn.microsoft.com/en-us/graph/auth-register-app-v2
Once you have registered your app, you need to get its client ID and client secret, and store them in secure variables:
$clientId = "your-app-client-id"
$clientSecret = ConvertTo-SecureString "your-app-client-secret" -AsPlainText -Force
Then, you need to connect to the Graph API using these credentials:
Connect-MgGraph -ClientId $clientId -ClientSecret $clientSecret -TenantId "your-tenant-id"
Now you are ready to retrieve Azure AD logs using the following cmdlets:
Get-MgAuditLogDirectoryAudit: This cmdlet returns the directory audit logs, which contain information about every task performed in your tenant, such as user creation, group membership changes, app consent grants, etc.
Get-MgAuditLogSignIn: This cmdlet returns the sign-in logs, which contain information about every sign-in attempt of your users, such as sign-in status, location, device, app, etc.
Both cmdlets support various parameters to filter and sort the results based on different criteria. For example, you can use the -Filter parameter to specify a condition that must be met by the log entries. You can use the -Top parameter to limit the number of results returned. You can use the -OrderBy parameter to sort the results by a specific property. You can use the -Select parameter to choose which properties to display.
Here are some examples of using these cmdlets:
Get the last 100 directory audit events
Get-MgAuditLogDirectoryAudit -Top 100
Get the directory audit events for a specific user
Get-MgAuditLogDirectoryAudit -Filter "userDisplayName eq 'Isabella Simonsen'"
Get the directory audit events for a specific activity
Get-MgAuditLogDirectoryAudit -Filter "activityDisplayName eq 'Add member to role'"
Get the last 100 sign-in events
Get-MgAuditLogSignIn -Top 100
Get the sign-in events for a specific user
Get-MgAuditLogSignIn -Filter "userDisplayName eq 'Isabella Simonsen'"
Get the sign-in events for a specific app
Get-MgAuditLogSignIn -Filter “appDisplayName eq ‘Microsoft Teams'"
Get the sign-in events with a specific status
Get-MgAuditLogSignIn -Filter “status/errorCode eq 0"
Get the sign-in events sorted by creation date
Get-MgAuditLogSignIn -OrderBy "createdDateTime desc"
Get only the relevant properties of sign-in events
Get-MgAuditLogSignIn -Select "userDisplayName","appDisplayName","location","status","createdDateTime"
You can also use PowerShell operators and expressions to further manipulate and analyze the results. For example, you can use the Where-Object cmdlet to filter the results based on a custom condition. You can use the Group-Object cmdlet to group the results by a common property. You can use the Measure-Object cmdlet to calculate statistics on numeric properties. You can use the Export-Csv cmdlet to export the results to a CSV file.
Here are some examples of using these operators and expressions:
Get the sign-in events with an unknown or risky location
Get-MgAud
itLogSignIn | Where-Object {$_.location.city -eq “unknown” -or $_.location