Working with Microsoft Graph API with JavaScript
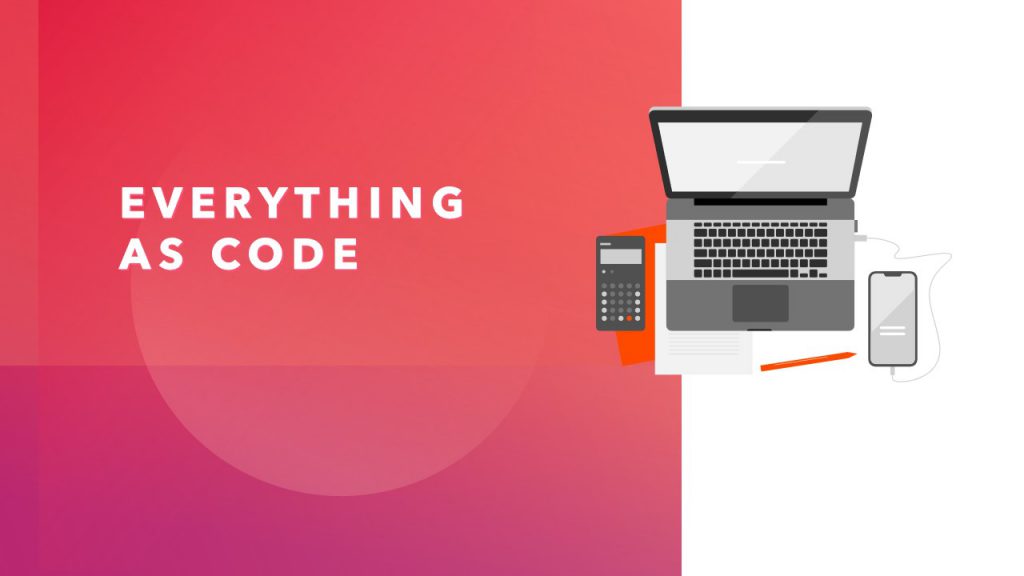
The Microsoft Graph API is a RESTful web API that enables you to access Microsoft Cloud service resources. You can use the Microsoft Graph API with JavaScript to build applications that authenticate with the Microsoft identity platform and perform actions on data in Microsoft 365, such as reading and writing to user mailboxes, managing groups, and working with OneDrive files.
To use the Microsoft Graph API with JavaScript, you’ll need to do the following:
- Register your application with Azure AD. This will give you a client ID and a client secret, which you’ll use to authenticate your application with the Microsoft identity platform.
- Install the Microsoft Graph JavaScript client library using npm (the Node Package Manager).
- Authenticate your application with the Microsoft identity platform using the client ID and client secret that you obtained in step 1.
- Make requests to the Microsoft Graph API using the Microsoft Graph JavaScript client library. You can use the library to construct HTTP requests and parse the responses from the API.
Here’s an example of how you might use the Microsoft Graph JavaScript client library to get the current user’s profile information:
import { Client } from '@microsoft/microsoft-graph-client';
async function getProfile() {
// Initialize the Graph client
const client = Client.init({
authProvider: (done) => {
// TODO: Add authentication code here
}
});
// Get the current user's profile
const user = await client.api('/me').get();
console.log(user);
}
For more information and examples of using the Microsoft Graph API with JavaScript, you can refer to the Microsoft Graph documentation.